Tipos De Variables Dev C++
Tabla De Tipos De Variables En Dev C++
Hay dos clases de tipos en C#: tipos de valor y tipos de referencia.There are two kinds of types in C#: value types and reference types.Las variables de tipos de valor contienen directamente los datos, mientras que las variables de los tipos de referencia almacenan referencias a los datos, lo que se conoce como objetos.Variables of value types directly contain their data whereas variables of reference types store references to their data, the latter being known as objects.Con los tipos de referencia, es posible que dos variables hagan referencia al mismo objeto y que, por tanto, las operaciones en una variable afecten al objeto al que hace referencia la otra.With reference types, it's possible for two variables to reference the same object and thus possible for operations on one variable to affect the object referenced by the other variable.Con los tipos de valor, cada variable tiene su propia copia de los datos y no es posible que las operaciones en una variable afecten a la otra (excepto para las variables de parámetro ref
y out
).With value types, the variables each have their own copy of the data, and it isn't possible for operations on one to affect the other (except for ref
and out
parameter variables).
Los tipos de valor de C# se dividen en tipos simples, tipos de enumeración, tipos de estructura y tipos de valores NULL.C#’s value types are further divided into simple types, enum types, struct types, and nullable value types.Los tipos de referencia de C# se dividen en tipos de clase, tipos de interfaz, tipos de matriz y tipos delegados.C#’s reference types are further divided into class types, interface types, array types, and delegate types.
En el esquema siguiente se proporciona información general del sistema de tipos de C#.The following outline provides an overview of C#’s type system.
Para definir variables de tipo cadena, estas se definen como vectores de caracteres, esto es, anteponiendo la palabra reservada char al identificador de la variable. Por defecto, Dev-C crea el archivo main.c, pero lo borramos ya que queremos aprender a programar desde el principio. Feb 12, 2020 🎯 Tutorial Variables y Tipos de Datos en C con DEV C 2020 👨🏿💻. Variables and types The usefulness of the 'Hello World' programs shown in the previous chapter is rather questionable. We had to write several lines of code, compile them, and then execute the resulting program, just to obtain the result of a simple sentence written on the screen.
- Tipos de valorValue types
- Tipos simplesSimple types
- Entero con signo:
sbyte
,short
,int
,long
Signed integral:sbyte
,short
,int
,long
- Entero sin signo:
byte
,ushort
,uint
,ulong
Unsigned integral:byte
,ushort
,uint
,ulong
- Caracteres Unicode:
char
Unicode characters:char
- Punto flotante binario IEEE:
float
,double
IEEE binary floating-point:float
,double
- Punto flotante decimal de alta precisión:
decimal
High-precision decimal floating-point:decimal
- Booleano:
bool
Boolean:bool
- Entero con signo:
- Tipos de enumeraciónEnum types
- Tipos definidos por el usuario con el formato
enum E {..}
User-defined types of the formenum E {..}
- Tipos definidos por el usuario con el formato
- Tipos de estructuraStruct types
- Tipos definidos por el usuario con el formato
struct S {..}
User-defined types of the formstruct S {..}
- Tipos definidos por el usuario con el formato
- Tipos de valores que aceptan valores NULLNullable value types
- Extensiones de todos los demás tipos de valor con un valor
null
Extensions of all other value types with anull
value
- Extensiones de todos los demás tipos de valor con un valor
- Tipos simplesSimple types
- Tipos de referenciaReference types
- Tipos de claseClass types
- Clase base definitiva de todos los demás tipos:
object
Ultimate base class of all other types:object
- Cadenas Unicode:
string
Unicode strings:string
- Tipos definidos por el usuario con el formato
class C {..}
User-defined types of the formclass C {..}
- Clase base definitiva de todos los demás tipos:
- Tipos de interfazInterface types
- Tipos definidos por el usuario con el formato
interface I {..}
User-defined types of the forminterface I {..}
- Tipos definidos por el usuario con el formato
- Tipos de matrizArray types
- Unidimensional y multidimensional; por ejemplo,
int[]
yint[,]
Single- and multi-dimensional, for example,int[]
andint[,]
- Unidimensional y multidimensional; por ejemplo,
- Tipos delegadosDelegate types
- Tipos definidos por el usuario con el formato
delegate int D(..)
User-defined types of the formdelegate int D(..)
- Tipos definidos por el usuario con el formato
- Tipos de claseClass types
Para obtener más información sobre los tipos numéricos, vea Tipos enteros y Tabla de tipos de punto flotante.For more information about numeric types, see Integral types and Floating-point types table.
El tipo bool
de C# se utiliza para representar valores booleanos; valores que son true
o false
.C#’s bool
type is used to represent Boolean values—values that are either true
or false
.
El procesamiento de caracteres y cadenas en C# utiliza la codificación Unicode.Character and string processing in C# uses Unicode encoding.El tipo char
representa una unidad de código UTF-16 y el tipo string
representa una secuencia de unidades de código UTF-16.The char
type represents a UTF-16 code unit, and the string
type represents a sequence of UTF-16 code units.
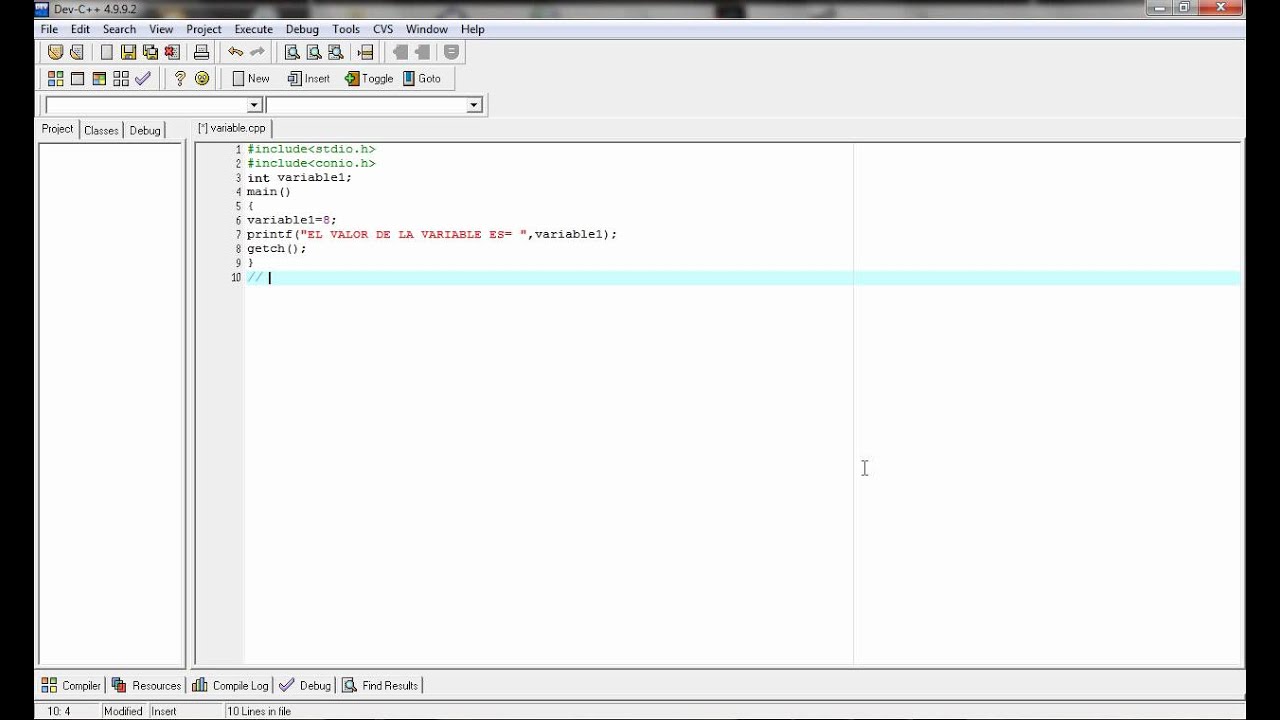
Tipos De Variables Estadisticas
Los programas de C# utilizan declaraciones de tipos para crear nuevos tipos.C# programs use type declarations to create new types.Una declaración de tipos especifica el nombre y los miembros del nuevo tipo.A type declaration specifies the name and the members of the new type.Cinco de las categorías de tipos de C# las define el usuario: tipos de clase, tipos de estructura, tipos de interfaz, tipos de enumeración y tipos delegados.Five of C#’s categories of types are user-definable: class types, struct types, interface types, enum types, and delegate types.
A tipo class
define una estructura de datos que contiene miembros de datos (campos) y miembros de función (métodos, propiedades y otros).A class
type defines a data structure that contains data members (fields) and function members (methods, properties, and others).Los tipos de clase admiten herencia única y polimorfismo, mecanismos por los que las clases derivadas pueden extender y especializar clases base.Class types support single inheritance and polymorphism, mechanisms whereby derived classes can extend and specialize base classes.
Un tipo struct
es similar a un tipo de clase, por el hecho de que representa una estructura con miembros de datos y miembros de función.A struct
type is similar to a class type in that it represents a structure with data members and function members.Pero a diferencia de las clases, las estructuras son tipos de valor y no suelen requerir la asignación del montón.However, unlike classes, structs are value types and don't typically require heap allocation.Los tipos de estructura no admiten la herencia especificada por el usuario y todos se heredan implícitamente del tipo object
.Struct types don't support user-specified inheritance, and all struct types implicitly inherit from type object
.
Un tipo interface
define un contrato como un conjunto con nombre de miembros de función públicos.An interface
type defines a contract as a named set of public function members.Un class
o struct
que implementa un interface
debe proporcionar implementaciones de miembros de función de la interfaz.A class
or struct
that implements an interface
must provide implementations of the interface’s function members.Un interface
puede heredar de varias interfaces base, y un class
o struct
pueden implementar varias interfaces.An interface
may inherit from multiple base interfaces, and a class
or struct
may implement multiple interfaces.
Un tipo delegate
representa las referencias a métodos con una lista de parámetros determinada y un tipo de valor devuelto.A delegate
type represents references to methods with a particular parameter list and return type.Los delegados permiten tratar métodos como entidades que se puedan asignar a variables y se puedan pasar como parámetros.Delegates make it possible to treat methods as entities that can be assigned to variables and passed as parameters.Los delegados son análogos a los tipos de función proporcionados por los lenguajes funcionales.Delegates are analogous to function types provided by functional languages.También son similares al concepto de punteros de función de otros lenguajes.They're also similar to the concept of function pointers found in some other languages.A diferencia de los punteros de función, los delegados están orientados a objetos y tienen seguridad de tipos.Unlike function pointers, delegates are object-oriented and type-safe.
Los tipos class
, struct
, interface
y delegate
admiten parámetros genéricos, mediante los que se pueden parametrizar con otros tipos.The class
, struct
, interface
, and delegate
types all support generics, whereby they can be parameterized with other types.
Tipos De Variables Programacion
Un tipo enum
es un tipo distinto con constantes con nombre.An enum
type is a distinct type with named constants.Cada tipo enum
tiene un tipo subyacente, que debe ser uno de los ocho tipos enteros.Every enum
type has an underlying type, which must be one of the eight integral types.El conjunto de valores de un tipo enum
es igual que el conjunto de valores del tipo subyacente.The set of values of an enum
type is the same as the set of values of the underlying type.
Tipos De Variables En Vba
C# admite matrices unidimensionales y multidimensionales de cualquier tipo.C# supports single- and multi-dimensional arrays of any type.A diferencia de los tipos enumerados antes, no es necesario declarar los tipos de matriz antes de usarlos.Unlike the types listed above, array types don't have to be declared before they can be used.En su lugar, los tipos de matriz se crean mediante un nombre de tipo entre corchetes.Instead, array types are constructed by following a type name with square brackets.Por ejemplo, int[]
es una matriz unidimensional de int
, int[,]
es una matriz bidimensional de int
y int[][]
es una matriz unidimensional de la matriz unidimensional de int
.For example, int[]
is a single-dimensional array of int
, int[,]
is a two-dimensional array of int
, and int[][]
is a single-dimensional array of single-dimensional array of int
.
Tampoco es necesario declarar los tipos que admiten un valor NULL antes de usarlos.Nullable value types also don't have to be declared before they can be used.Para cada tipo de valor T
que no acepta valores NULL, existe un tipo de valor T?
que admite un valor NULL correspondiente, que puede tener un valor adicional, null
.For each non-nullable value type T
, there is a corresponding nullable value type T?
, which can hold an additional value, null
.Por ejemplo, int?
es un tipo que puede contener cualquier número entero de 32 bits o el valor null
.For instance, int?
is a type that can hold any 32-bit integer or the value null
.
Dev c won't compile. El sistema de tipos de C# está unificado, de tal forma que un valor de cualquier tipo puede tratarse como un object
.C#’s type system is unified such that a value of any type can be treated as an object
.Todos los tipos de C# directa o indirectamente se derivan del tipo de clase object
, y object
es la clase base definitiva de todos los tipos.Every type in C# directly or indirectly derives from the object
class type, and object
is the ultimate base class of all types.Los valores de tipos de referencia se tratan como objetos mediante la visualización de los valores como tipo object
.Values of reference types are treated as objects simply by viewing the values as type object
.Los valores de tipos de valor se tratan como objetos mediante la realización de operaciones de conversión boxing y operaciones de conversión unboxing.Values of value types are treated as objects by performing boxing and unboxing operations.En el ejemplo siguiente, un valor int
se convierte en object
y vuelve a int
.In the following example, an int
value is converted to object
and back again to int
.
Cuando se convierte un valor de un tipo de valor al tipo object
, se asigna una instancia object
, también denominada 'box', para contener el valor, y el valor se copia en dicho box.When a value of a value type is converted to type object
, an object
instance, also called a 'box', is allocated to hold the value, and the value is copied into that box.Por el contrario, cuando se convierte una referencia object
en un tipo de valor, se comprueba si la referencia object
es un box del tipo de valor correcto y, si la comprobación es correcta, se copia el valor del box.Conversely, when an object
reference is cast to a value type, a check is made that the referenced object
is a box of the correct value type, and, if the check succeeds, the value in the box is copied out.
El sistema de tipos unificado de C# conlleva efectivamente que los tipos de valor pueden convertirse en objetos 'a petición'.C#’s unified type system effectively means that value types can become objects 'on demand.'Debido a la unificación, las bibliotecas de uso general que utilizan el tipo object
pueden usarse con tipos de referencia y tipos de valor.Because of the unification, general-purpose libraries that use type object
can be used with both reference types and value types.
Hay varios tipos de variables en C#, entre otras, campos, elementos de matriz, variables locales y parámetros.There are several kinds of variables in C#, including fields, array elements, local variables, and parameters.Las variables representan ubicaciones de almacenamiento, y cada variable tiene un tipo que determina qué valores pueden almacenarse en la variable, como se muestra a continuación.Variables represent storage locations, and every variable has a type that determines what values can be stored in the variable, as shown below.
- Tipo de valor distinto a NULLNon-nullable value type
- Un valor de ese tipo exactoA value of that exact type
- Tipos de valor NULLNullable value type
- Un valor
null
o un valor de ese tipo exactoAnull
value or a value of that exact type
- Un valor
- objetoobject
- Una referencia
null
, una referencia a un objeto de cualquier tipo de referencia o una referencia a un valor de conversión boxing de cualquier tipo de valorAnull
reference, a reference to an object of any reference type, or a reference to a boxed value of any value type
- Una referencia
- Tipo de claseClass type
- Una referencia
null
, una referencia a una instancia de ese tipo de clase o una referencia a una instancia de una clase derivada de ese tipo de claseAnull
reference, a reference to an instance of that class type, or a reference to an instance of a class derived from that class type
- Una referencia
- Tipo de interfazInterface type
- Un referencia
null
, una referencia a una instancia de un tipo de clase que implementa dicho tipo de interfaz o una referencia a un valor de conversión boxing de un tipo de valor que implementa dicho tipo de interfazAnull
reference, a reference to an instance of a class type that implements that interface type, or a reference to a boxed value of a value type that implements that interface type
- Un referencia
- Tipo de matrizArray type
- Una referencia
null
, una referencia a una instancia de ese tipo de matriz o una referencia a una instancia de un tipo de matriz compatibleAnull
reference, a reference to an instance of that array type, or a reference to an instance of a compatible array type
- Una referencia
- Tipo delegadoDelegate type
- Una referencia
null
o una referencia a una instancia de un tipo delegado compatibleAnull
reference or a reference to an instance of a compatible delegate type
- Una referencia